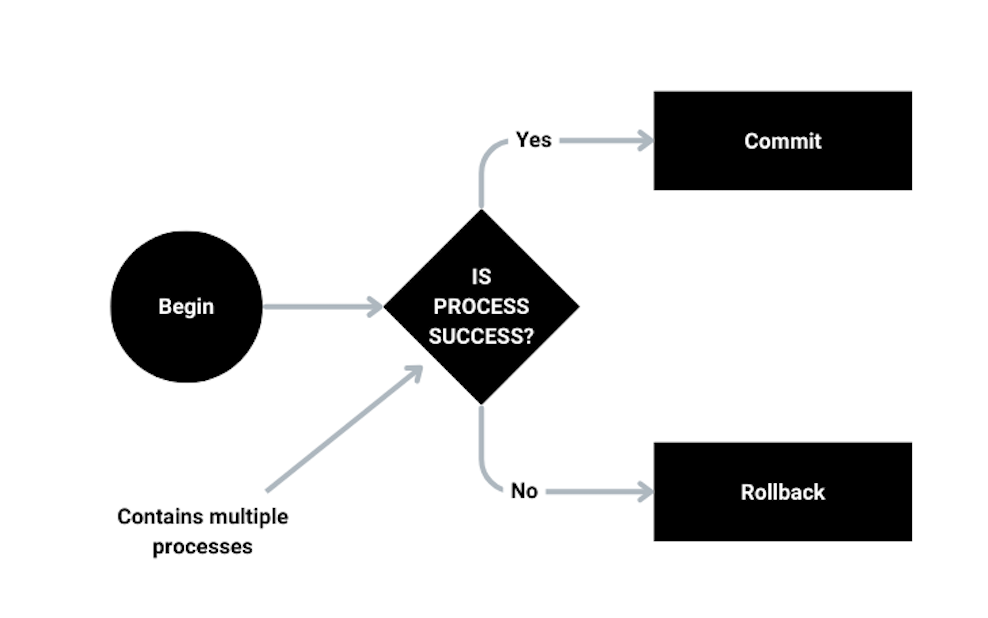
Understanding Database Transactions in Laravel
#database
#transaction
#laravel
Imagine you’re building an e-commerce application where a customer places an order. This process involves multiple database operations: reducing inventory count, creating an order record, processing payment, and updating the customer’s purchase history. What happens if the payment processing fails after the inventory has already been reduced? Without proper safeguards, you’d end up with inconsistent data - products missing from inventory without a corresponding successful order.
This is where database transactions come in to save the day.
What is a Database Transaction?
A database transaction is a logical unit of work that contains one or more database operations (such as inserts, updates, or deletes). The key characteristic of transactions is that they follow the ACID properties:
- Atomicity: All operations within a transaction succeed or fail together. If any operation fails, the entire transaction is rolled back.
- Consistency: A transaction brings the database from one valid state to another valid state.
- Isolation: Transactions operate independently of each other. Incomplete transactions remain invisible to other transactions.
- Durability: Once a transaction is committed, its effects remain permanent even in the case of system failure.
In simple terms, transactions ensure that your database operations are reliable, consistent, and safe even when errors occur.
Database Transactions in Laravel
Laravel, a popular PHP framework, provides an elegant way to work with database transactions through its database query builder and Eloquent ORM. Laravel’s transaction methods abstract away the complexity of managing database transactions manually.
You can use transactions in Laravel through several methods:
1. Using the DB Facade
use Illuminate\Support\Facades\DB;
DB::transaction(function () {
// Multiple database operations here
DB::table('products')->where('id', 1)->decrement('stock', 1);
DB::table('orders')->insert([
'product_id' => 1,
'user_id' => Auth::id(),
'quantity' => 1,
// other order details
]);
});
In this example, if any exception occurs within the closure, Laravel automatically rolls back the transaction. If everything executes successfully, the transaction is committed.
2. Manual Transaction Control
use Illuminate\Support\Facades\DB;
try {
DB::beginTransaction();
// Multiple database operations
$product = Product::find(1);
$product->stock -= 1;
$product->save();
$order = new Order();
$order->product_id = 1;
$order->user_id = Auth::id();
$order->quantity = 1;
$order->save();
// If we reach here, everything succeeded
DB::commit();
} catch (\Exception $e) {
// Something went wrong, rollback the transaction
DB::rollBack();
// You might want to log the error or notify someone
Log::error('Order failed: ' . $e->getMessage());
// Rethrow or handle the exception as needed
throw $e;
}
3. Transaction with Return Value
$order = DB::transaction(function () {
$product = Product::lockForUpdate()->find(1);
$product->stock -= 1;
$product->save();
$order = Order::create([
'product_id' => $product->id,
'user_id' => Auth::id(),
'quantity' => 1,
'total' => $product->price,
]);
return $order;
});
This approach is useful when you need to return a value from your transaction closure.
Best Practices for Using Transactions
- Keep transactions as short as possible to reduce lock contention
- Only include operations that need to be atomic
- Be mindful of deadlocks in high-concurrency situations
- Consider using
lockForUpdate()
when you need to prevent race conditions - Handle exceptions properly to ensure transactions are rolled back when errors occur
By properly implementing database transactions in your Laravel applications, you can ensure data integrity even when operations fail unexpectedly, leading to more robust and reliable applications.