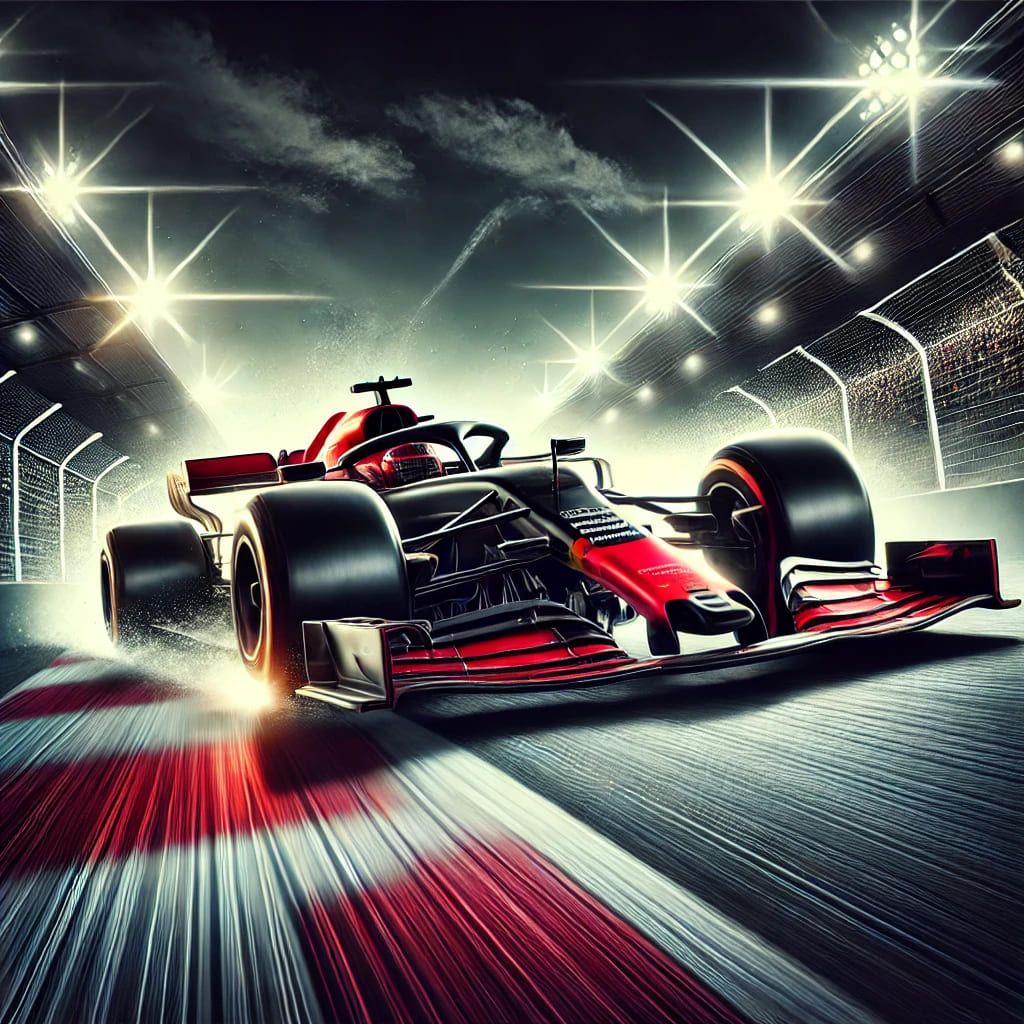
Preventing Race Condition in Laravel
#database
#race condition
#laravel
Real-Life Example of Race Condition
Let’s consider a common scenario in an e-commerce system where two customers attempt to purchase the last available item simultaneously. Both customers check the inventory (showing 1 item), add it to their cart, and proceed to checkout. Without proper synchronization, both transactions might successfully complete, leading to overselling and a negative inventory count. This situation perfectly demonstrates how race conditions can create data inconsistency in real-world applications.
What is Race Condition?
A race condition is a software bug that occurs when the timing or order of events affects the correctness of a program. It happens when multiple processes or threads try to access and manipulate shared resources simultaneously without proper synchronization.
Race conditions can lead to:
- Data corruption
- Unexpected behavior
- System crashes
- Security vulnerabilities
Handling Race Conditions in Laravel
Laravel provides several methods to handle race conditions:
1. Database Transactions
DB::transaction(function () {
// Your code here
// If any exception occurs, all operations will be rolled back
});
2. Pessimistic Locking
Product::lockForUpdate()->find(1);
// This prevents other transactions from reading or writing to the selected rows
Example
As documented in Laravel’s Pessimistic Locking section, you should wrap pessimistic locks within a transaction. This ensures the data remains unaltered in the database until the entire operation is complete.
try {
$order = \DB::transaction(function () use ($request) {
// Lock the row for update
$product = \App\Models\Product::lockForUpdate()
->find($request->input('product_id'));
info('Current product', ['product' => $product->toArray()]);
if ($product->stock < 1) {
throw new \RuntimeException('Out of stock');
} else {
$product->stock -= 1;
$product->save();
}
// Create an order
return \App\Models\Order::create([
'user_id' => $request->input('user_id'),
'product_id' => $product->id,
]);
});
} catch (\Throwable $e) {
report($e);
return response()->json([
'message' => $e->getMessage(),
], 400);
}
return response()->json([
'message' => 'Order created',
'order' => $order,
]);
Important note: lockForUpdate()
only works within a transaction. If you try to use it outside a transaction, it won’t provide any locking benefits and could lead to race conditions.
Testing Race Conditions with Fork
To demonstrate and test race conditions in Laravel, we can use the Fork package by Spatie. This package allows us to run PHP code concurrently, making it easier to simulate race conditions in a controlled environment.
$results = \Spatie\Fork\Fork::new()
->run(
function () {
return Http::post(route('order'), [
'product_id' => 1,
'user_id' => 1,
])->body();
},
function () {
return Http::post(route('order'), [
'product_id' => 1,
'user_id' => 2,
])->body();
}
);
In this code example, we’re simulating two concurrent requests to create orders for the same product. Without proper locking mechanisms, both requests might succeed even if there’s only one item in stock. However, with our previous implementation using pessimistic locking, one request will succeed while the other will receive an “Out of stock” error.
The API receives product_id
and user_id
parameters. While user_id
should normally be resolved through a session or token, it will be hardcoded in this scenario. For our test case, we’ll use product ID 1 (an iPhone 16) with a stock quantity of 1. Our expected outcomes are:
- The iPhone product stock is reduced to 0
- Only one order is successfully created
Test Results Using Locking
[2025-03-09 07:51:28] local.INFO: Current product {"product":{"id":1,"name":"Iphone 16","stock":1,"created_at":"2025-03-09T07:51:17.000000Z","updated_at":"2025-03-09T07:51:17.000000Z"}}
[2025-03-09 07:51:28] local.INFO: Current product {"product":{"id":1,"name":"Iphone 16","stock":0,"created_at":"2025-03-09T07:51:17.000000Z","updated_at":"2025-03-09T07:51:28.000000Z"}}
[2025-03-09 07:51:28] local.INFO: Result 1 {"message":"Order created","order":{"user_id":1,"product_id":1,"updated_at":"2025-03-09T07:51:28.000000Z","created_at":"2025-03-09T07:51:28.000000Z","id":1}}
[2025-03-09 07:51:28] local.INFO: Result 2 {"message":"Out of stock"}
From the results above, we can see that the first request was successfully created, while the second request failed. The logs also show that both requests were executed at the exact same hour, minute, and second.
Test Results Without Locking
[2025-03-09 08:04:06] local.INFO: Current product {"product":{"id":1,"name":"Iphone 16","stock":1,"created_at":"2025-03-09T08:03:49.000000Z","updated_at":"2025-03-09T08:03:49.000000Z"}}
[2025-03-09 08:04:06] local.INFO: Current product {"product":{"id":1,"name":"Iphone 16","stock":1,"created_at":"2025-03-09T08:03:49.000000Z","updated_at":"2025-03-09T08:03:49.000000Z"}}
[2025-03-09 08:04:07] local.INFO: Result 1 {"message":"Order created","order":{"user_id":1,"product_id":1,"updated_at":"2025-03-09T08:04:06.000000Z","created_at":"2025-03-09T08:04:06.000000Z","id":1}}
[2025-03-09 08:04:07] local.INFO: Result 2 {"message":"Order created","order":{"user_id":2,"product_id":1,"updated_at":"2025-03-09T08:04:06.000000Z","created_at":"2025-03-09T08:04:06.000000Z","id":2}}
From the test results above without using locks, we can see that both requests successfully created orders and accessed the product data simultaneously. This resulted in the iPhone 16’s stock becoming 0, which should not have happened. This demonstrates the importance of using locking mechanisms to prevent race conditions in e-commerce applications.
You can find a complete example demonstrating race conditions and their prevention in Laravel in this repository